Issue #461
With UIAlertController we can add buttons and textfields, and just that
func addAction(UIAlertAction)
func addTextField(configurationHandler: ((UITextField) -> Void)?)
To add custom content to UIAlertController, there are some workarounds
Add content onto UITextField
Restyle UITextField
and add custom content
Subclass UIAlertController and handle UI in viewWillAppear
By subclassing we can tweak the whole view hierarchy and listen to events like layout subviews, but this can be very fragile.
Make custom UIViewController that looks like UIAlertController
This is the correct way but takes too much time to imitate UIAlertController, and have to deal with UIVisualEffectView, resize view for different screen sizes and dark mode
Add view to UIAlertController view
We can detect UILabel
to add our custom view below it, using How to find subview recursively in Swift
But a less work approach is to add it above buttons, this is easily achieved by offsetting bottom.
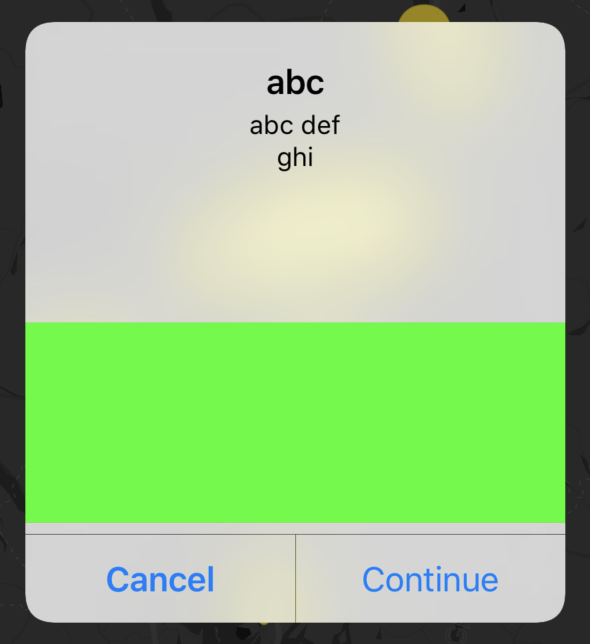
The trick is here is to have line breaks in message to leave space for our custom view.
let controller = UIAlertController(
title: title,
message: message + String(repeating: "\n", count: 10),
preferredStyle: .alert
)
let continueAction = UIAlertAction(
title: R.string.localizable.continue(),
style: .default) { _ in
action()
}
let cancelAction = UIAlertAction(title: R.string.localizable.cancel(), style: .cancel)
controller.addAction(cancelAction)
controller.addAction(continueAction)
let customView = UIView()
customView.backgroundColor = UIColor.green
controller.view.addSubview(customView)
NSLayoutConstraint.on([
customView.leftAnchor.constraint(equalTo: controller.view.leftAnchor),
customView.rightAnchor.constraint(equalTo: controller.view.rightAnchor),
customView.bottomAnchor.constraint(equalTo: controller.view.bottomAnchor, constant: -50),
customView.heightAnchor.constraint(equalToConstant: 100)
])
Start the conversation