Issue #820
Use NSDataDetector
to detect links, and NSMutableAttributedString
to mark link range. Then we enumerateAttributes
and build our Text
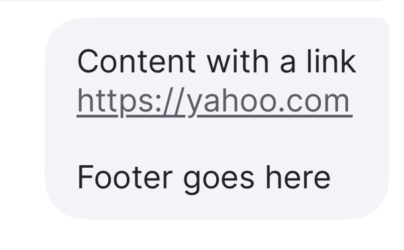
func attribute(string: String) -> Text {
guard let detector = try? NSDataDetector(types: NSTextCheckingResult.CheckingType.link.rawValue)
else {
return Text(string)
}
let stringRange = NSRange(location: 0, length: string.count)
let matches = detector.matches(
in: string,
options: [],
range: stringRange
)
let attributedString = NSMutableAttributedString(string: string)
for match in matches {
attributedString.addAttribute(.underlineStyle, value: NSUnderlineStyle.single, range: match.range)
}
var text = Text("")
attributedString.enumerateAttributes(in: stringRange, options: []) { attrs, range, _ in
var t = Text(attributedString.attributedSubstring(from: range).string)
if attrs[.underlineStyle] != nil {
t = t
.foregroundColor(.gray)
.underline()
} else {
t = t
.foregroundColor(Color(UIColor.label))
}
text = text + t
}
return text
}
Start the conversation